Charging Credit Cards using an iFrame
PCI Compliance is the security implemented for the credit card industry. Use Pay Advantages iFrame to ensure you remain PCI compliant.
Capturing cards using our iframe is the most secure process to capture and store cards thereby limiting your PCI exposure (PCI-DSS is a Payment Card Industry Data Security Standard for managing credit cards so they do not get leaked into the wrong hands). Using the iframe ensures credit card information is captured and stored directly in our secure PCI compliant Card Data Environment.
Pay Advantage hosts a Javascript library which simplifies the consumption of the Pay Advantage Credit Card iframe.
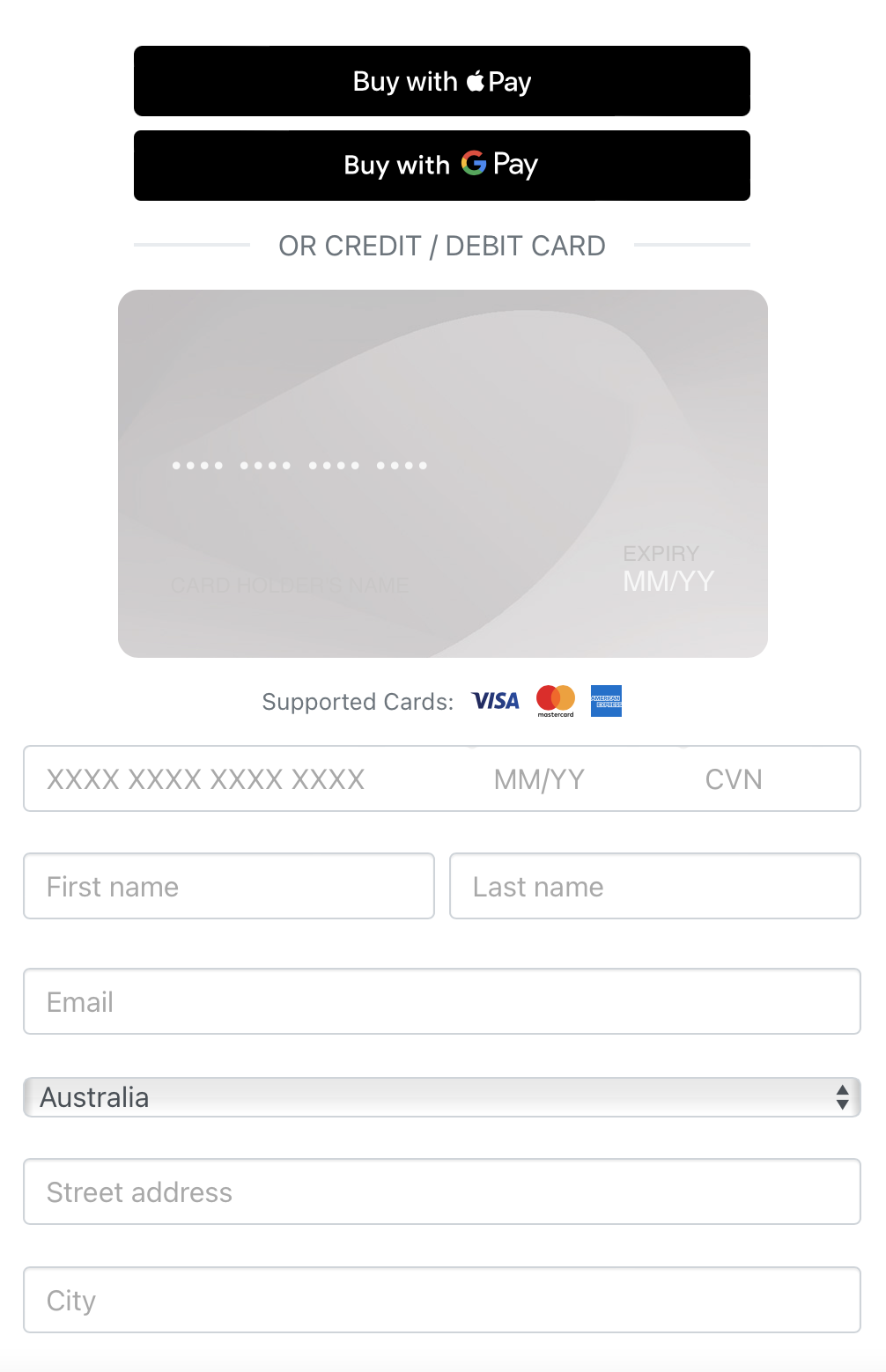
Embed the above Pay Advantage iframe into your website to facilitate PCI secure credit card payments.
To integrate the iframe into your checkout page follow the below steps:
Step 1: Prepare your page
Add the following resources to your page:
<link rel="stylesheet" text="text/css"
href="https://api.payadvantage.com.au/v3/creditcardcapture.css" />
<script src="https://api.payadvantage.com.au/v3/creditcardcapture.js" />
Step 2: Create an iframe placeholder on your web application.
The CSS class in the iframe code block below is used by the CSS script resource imported above to control the height and width of the iframe so that it doesn’t show borders or scroll bars.
<iframe class="pay-advantage-credit-card-capture" src="" >
</iframe>
Step 3: Get a Unique iframe Url
Using the Pay Advantage API, make a call to obtain an iframe Url to be consumed by your page. This Url will contain single-use NONCE token that verifies you have given permission for this iframe to be used on your account.
This step must be completed by your backend server. You will need to create an API call from your front end to the back end to return the iframe URL. This is because the URL /credit_card_iframe
endpoint requires your Pay Advantage bearer token which should always be kept safe in your backend. It is not safe to handle this API request directly using the browser.
This creates a URL that initialises the Credit Card Capture IFrame for use. This URL is short lived and should be used to initialise the PayAdvantageCreditCardCapture once it is available.
Make the IFrameUrl property of the response available to your page.
API CALL POST: v3/credit_card_iframes
Type | Required | Parameter Name | Description |
---|---|---|---|
Object | Y | Customer | The customer code who owns the credit card. |
Number | Y | Amount | The amount of the payment. Set this to 0.00 to tokenise a card without charging it. |
String | Y | Description | The description of the payment. When the amount is 0.00, this field is ignored |
Boolean | N | OnchargeFees | The desired intent of the payment and if the fee amount should be on charged. Default is: false |
String | N | ExternalID | Your identifier for the payment. |
Object | N | CustomerPaymentAccount | The customer payment to use for a pre-filled card purchase. Object contains Code and CanChange. CanChange indicates if the customer is able to process a different card, Code is the CustomerPaymentAccount code. |
Example with no pre-filled card:
POST: <https://api.payadvantage.com.au/v3/credit_card_iframes>
{
"Customer:{
"Code":"ABC123"
},
"Amount": 9000.00,
"OnChargeFees: true,
"Description": "Joining fee",
"ExternalID": "7871882773"
}
Response
Content-Type: application/json
{
"IFrameUrl":".../index.html?nonce=XXXX&nonce_type=credit_card_iframe"
}
Example with pre-tokenized card:
POST: <https://api.payadvantage.com.au/v3/credit_card_iframes>
{
"Customer:{
"Code":"ABC123"
},
"Amount": 9000.00,
"OnChargeFees: true,
"Description": "Joining fee",
"ExternalID": "7871882773",
"CustomerPaymentAccount":{
"Code":"ABC123",
"CanChange":true
}
}
Response
Content-Type: application/json
{
"IFrameUrl":".../index.html?nonce=XXXX&nonce_type=credit_card_iframe"
}
Step 4: Create an Instance of PayAdvantageCreditCardCapture
On your page, create an instance of PayAdvantageCreditCardCapture for controlling your iframe.
var captureIframe = document.querySelector('.pay-advantage-credit-card-capture');
var creditCardCapture = new PayAdvantageCreditCardCapture();
creditCardCapture
.initialise(captureIframe, IFrameUrl, options, cardHolderDetails)
.then(function(result) {
// Any logic goes here related to the iframe loading.
})
.catch(function(error) {
console.log(error);
});
Note: Creating an instance of PayAdvantageCreditCardCapture attaches handlers to the window object. You should dispose of the PayAdvantageCreditCardCapture instance when no longer required to release the handlers.
creditCardCapture.dispose();
References
initialise
Initialise - Attaches an iframe and registers the iframe message listeners with the window object. Once initialised, the iframe is valid for 30 minutes. After that time, its security will have expired and it will need to be reloaded.
PayAdvantageCreditCardCapture.initialise(
iframeElement: HTMLIFrameElement,
url: string,
options?: {
showCard: true
},
cardHolderDetails?: {
firstName?: string,
lastName?: string,
email?: string,
address?: {
addressLine1?: string,
city?: string,
country?: string,
postcode?: string,
state?: string,
}
}
): Promise
Type | Required | Parameter Name | Description |
---|---|---|---|
PayAdvantageCreditCardCapture | Y | iframeElement | The iframe to attach this instance to (Step 4) |
String | Y | url | The IFrameUrl that was returned by a successful call to https://api.payadvantage.com.au/v3/credit_card_iframes (Step 3) |
Object | N | cardHolderDetails | Pre-fills the card holders details when payer authentication is used. All of the values are optional, and the system will prompt the user to complete them when necessary. |
addEventListener
PayAdvantageCreditCardCapture.addEventListener(
eventName: string,
listener: EventListenerOrEventListenerObject
): void
Parameter | Description | |
---|---|---|
eventName | The name of the event emitted from the iframe after certain actions. The currently supported option are: "change"- When a field on the form has been changed. "show-loader" – When a loading / busy overlay needs to be displayed and action buttons need to be disabled. "hide-loader" – When a loading / busy overlay can be removed and action buttons can be restored. "resize" – When the iframe changes size due to 3D Secure. "fee-calculated" - If on charge fee is true for the payment, this event will return the calculated fee with the following structure. Use totalAmount to show to the customer so they are aware of the incurred fees. { AttemptFee:0.2, AttemptFeeTax:0.02, Card:{IssuingCountry: "United States"}, Msf:1.17, MsfTax:0.12, TotalAmount:1.51 } "fee-calculating" - If on charge fee is true for the payment, this will return true when the iframe is determining the fee for the charge "fee-calculate-error" - If on charge fee is true for the payment. This will return the error that has been produced when trying to determine the fee has failed. The payment can still be completed if the fee calculation has errors, but you should inform your customer that this payment will incur fees "device-complete" - When a payment through Apple or Google is successfully processed, there's no need to execute the authorize function because the payment is already complete. In such cases, your main task is to manage the event that provides a charge response. This response is presented as a parameter object named AuthorizePaymentResponse, which is identical to the object you receive from calling PayAdvantageCreditCardCapture.authorize(). "device-error" - If an apple or google payment has been initiated, but received an error specific to the native paysheet or initialization, then this event is provided to allow an error to be displayed. | |
listener | The listener to call when the event is triggered |
removeEventListener
Removes an event listener that was added with addEventListener.
PayAdvantageCreditCardCapture.removeEventListener(
eventName: string,
listener: EventListenerOrEventListenerObject
): void
Parameter | Description | |
---|---|---|
eventName | The name of the event to stop listening for events. Currently supported options are "change", "show-loader", "hide-loader", "fee-calculating", "fee-calculate-error", "fee-calculated" and "resize". | |
listener | The listener that was used to reference the call before |
dispose
Cleans up the object and releases all event listeners. This should be called to clean up the iframe set up once it is no longer needed.
PayAdvantageCreditCardCapture.dispose(): void
Step 5: Capturing the Card Details
The capture process will capture the card details and store it as a Customer Payment Account for that customer.
if (!creditCardCapture.checkValidity()) {
return false;
}
creditCardCapture
.authorize()
.then(function(result) {
// Save the credit card code for later use,
// Or use it to charge a card.
})
.catch(function(error) {
console.log(error);
});
References
checkValidity
Determines if all credit card fields are complete and valid (ready to capture).
PayAdvantageCreditCardCapture.checkValidity(): boolean
authorize
Attempts to store the card details entered into the iframe and returns the response.
PayAdvantageCreditCardCapture.authorize(): Promise
Example of Payment
{
"code": "Y7D7SA",
"externalID": null,
"description": "Invoice 123",
"createdBy": "An Awesome App",
"dateCaptured": "2022-10-07T08:40:26.027+11:00",
"dateCreated": "2022-10-07T08:40:25.963+11:00",
"amountCaptured": 100,
"amountCapturedIncFees": 100,
"payment": {
"dateFailed": null,
"failReason": null,
"failCode": null,
"code": "Z7D7SA"
},
"dateFailed": null,
"failReason": null,
"failCode": null,
"cvnCheckResponse": "matched",
"isProcessing": false,
"customerPaymentAccount": {
//store this code to charge this card later using the charge API
"code": "D7D7SA",
"dateFailed": null
},
"isUndetermined": false
}
Example of Tokenisation
{
"code": "UTY7SA",
"externalID": null,
"description": "store card",
"createdBy": "An Awesome App",
"dateCaptured": null,
"dateCreated": "2022-10-07T08:58:40.387+11:00",
"amountCaptured": null,
"amountCapturedIncFees": null,
"payment": null,
"dateFailed": null,
"failReason": null,
"failCode": null,
"cvnCheckResponse": "matched",
"isProcessing": false,
"customerPaymentAccount": {
//store this code to charge this card later using the charge API
"code": "D7D7SA",
"dateFailed": null
},
"isUndetermined": false
}
You will need to store the customerPaymentAccount.code
so that you can charge
the card later using Charge a Stored/Tokenised Card.
JSDoc Documentation
For further documentation of the iframe objects, ultilise the JSDoc information provided in the creditcardcapture.js file located here: https://api.payadvantage.com.au/v3/creditcardcapture.js
Updated about 1 month ago